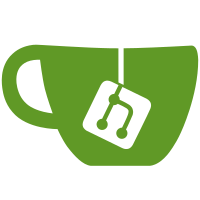
The idea here is to build cabal-helper with whatever version of Cabal the user happens to be using (which we find by looking at dist/setup-config) at runtime. This way we can support literally any version of Cabal as long as the actual cabal-helper still compiles. I tried to only use interfaces in Cabal that have been there since at least 1.16 so I'm hoping this shouldn't break too much.
42 lines
1.4 KiB
Haskell
42 lines
1.4 KiB
Haskell
-- ghc-mod: Making Haskell development *more* fun
|
|
-- Copyright (C) 2015 Daniel Gröber <dxld ÄT darkboxed DOT org>
|
|
--
|
|
-- This program is free software: you can redistribute it and/or modify
|
|
-- it under the terms of the GNU Affero General Public License as published by
|
|
-- the Free Software Foundation, either version 3 of the License, or
|
|
-- (at your option) any later version.
|
|
--
|
|
-- This program is distributed in the hope that it will be useful,
|
|
-- but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
-- MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
-- GNU Affero General Public License for more details.
|
|
--
|
|
-- You should have received a copy of the GNU Affero General Public License
|
|
-- along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
|
|
module Common where
|
|
|
|
import Control.Applicative
|
|
import Data.List
|
|
import Data.Maybe
|
|
import System.Environment
|
|
import System.IO
|
|
|
|
errMsg :: String -> IO ()
|
|
errMsg str = do
|
|
prog <- getProgName
|
|
hPutStrLn stderr $ prog ++ ": " ++ str
|
|
|
|
align :: String -> String -> String -> String
|
|
align n an str = let
|
|
h:rest = lines str
|
|
[hm] = match n h
|
|
rest' = [ move (hm - rm) r | r <- rest, rm <- match an r]
|
|
in
|
|
unlines (h:rest')
|
|
where
|
|
match p str' = maybeToList $
|
|
fst <$> find ((p `isPrefixOf`) . snd) ([0..] `zip` tails str')
|
|
move i str' | i > 0 = replicate i ' ' ++ str'
|
|
move i str' = drop i str'
|